Advanced mode gives you access to Name Mangler's Name Conversion Description Language (NCDL), which lets you describe a new filename as an expression (or series of expressions). Each expression may be one of the following:
- A literal
- A constant (i.e., a metadata placeholder)
- A function, possibly containing nested expressions
Most of the time, the new filename will be based on a [concatenate] function (see below) to combine the name you've chosen with an extension.
You can use regular expressions in Advanced mode. Before you do, though, you should be aware that Name Mangler uses Apple's built-in regular expression API in free-spacing mode. Please see the Settings help page for more information on the regex engine and how to disable free-spacing mode.
The Work Area
Selecting Advanced mode opens a mini text editor within Name Mangler.
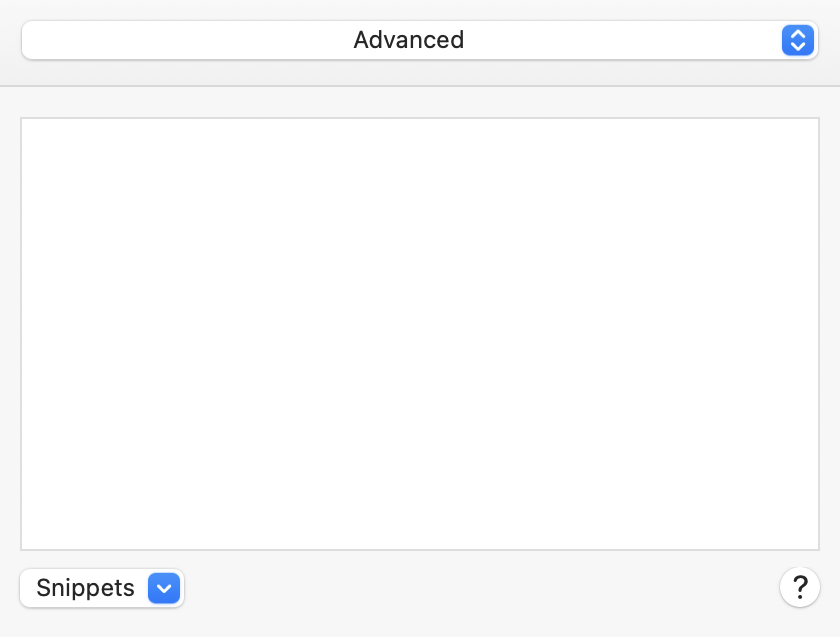
The open box is the text area, and it's got a few tricks up its sleeve to make working there easier:
Resize the editor area
To gain more work room, resize the edit area by dragging the divider bar that separates the file list area from the editor area.
Manage indents
Tab and Shift-Tab can be used to indent and outdent your text. After indenting, a newly created line will appear at the same location as the prior line.
You can change the indent (increase or decrease) on one or more rows by selecting the rows you wish to change, and then using Edit ➝ Shift Left (⌘[) and Edit ➝ Shift Right (⌘]).
Comment your work
Name Mangler ignores all text other than that within constants, functions, variables, and metadata. You can use this to your advantage to comment your code, i.e.:
[findRegularExpression "(a|b)" in <name> and replace with "c"]
The light gray text isn't read by Name Mangler at all, yet having it present makes it much simpler to understand what your code does. Comment liberally!
Take advantage of autocomplete
Metadata, variables, and functions can be autocompleted; type an opening angle bracket (for metadata and variables) or square bracket (for functions), and then start typing:
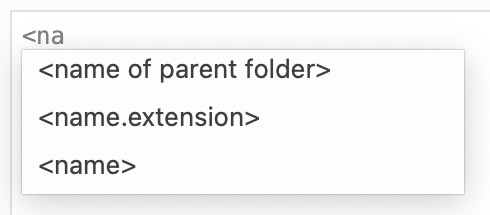
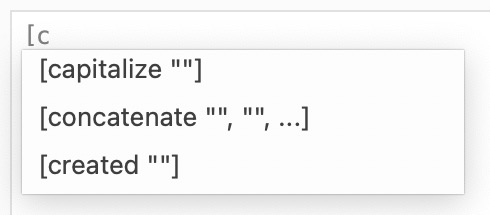
You can select one of the choices with a mouse click, or via the arrow keys. If you select a function, you'll see its parameters, as seen in "concatenate" in the above right image.
To select a highlighted item, either double-click it with the mouse, or press Return.
Snippets
Snippets are bits of code that can be reused—they can either be entire functional Advanced mode programs (though it may make more sense to save those as Presets). Name Mangler includes a few predefined snippets, and you can see these by clicking on the Snippets button at the bottom of the Advanced area's text input window:
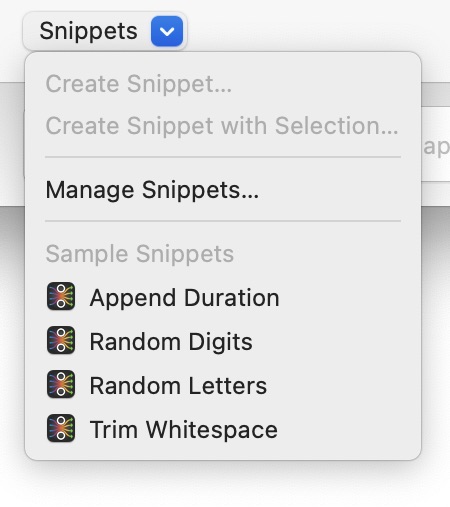
The provided snippets are simple and self-explanatory, so we won't cover them here. To use a snippet, just select it in the list, and it will be inserted at the cursor's present location in the edit window.
Using this menu, you can create a snippet from either the entire Advanced window (Create Snippet) or the currently selected text (Create Snippet with Selection). After creating a snippet, you'll be asked to give it a name, and from then on, it will be available in the pop-up menu.
To delete a snippet, hold down the Option key, then click the Snippets menu. Each existing snippet will be renamed to Move "name of snippet" to Trash. Select it, and that's exactly what will happen.
For mass snippet management (deleting all, renaming, etc.), select Manage Snippets to switch to the Snippets folder in the Finder.
Name Mangler can synchronize Snippets (and Presets) across multiple Macs. For more on how to set this up, see the Save Presets and Snippets in… section of the Settings help.
Literals
Literals are simply text surrounded by quotation marks, such as "Hawaii Pictures_" or "Skiing 2024 Utah". You can include any kind of Unicode text by putting it between quotation marks.
If you want the new filename to include quotation marks, then you will have to escape them with leading \ characters. For example, if you want your new filename to be My name is "File".txt, then the corresponding literal would be "My name is \"File\".txt"
Constants
Constant names must be surrounded by angle brackets, as in <some constant>. You can use any metadata field as a constant. Here are some examples:
Functions
Functions must be surrounded by square brackets, as in [someFunction]. NCDL does not look at anything but a given function's identifier (findRegularExpression in the following examples) to recognize it. Moreover, the sequence of arguments is not variable, although you are free to label these arguments however you like.
For instance, the following function calls will all lead to the same result:
- [findRegularExpression "(a|b)" in <name> and replace with "c"]
- [findRegularExpression "(a|b)" <name> "c"]
- [findRegularExpressions which are best described as "(a|b)" in the original file <name> and replace the previously mentioned regular expression with a simple "c" character]
- [findRegularExpression Labels are "(a|b)" hardly ever <name> relevant. "c" (Actually, there are a few exceptions to this rule…)]
- [findRegularExpression replacement: "(a|b)" regex: <name> source: "c"]
Quite obviously, the last example is misleading, and thus not recommended. As mentioned in example number four, there are a few cases where labels do make a difference. Please have a look at the (ignore case) feature in the [find] and [findRegularExpression] functions, and the (backwards) feature in the [insert] and [remove] functions for more information on that.
Note: Each top-level use of a function affects <name>. So if you string together a number of functions as top-level commands, as opposed to nesting functions, Name Mangler will run them sequentially. For example:
- [find "A" in <name> and replace with "1"]
- [find "B" in <name> and replace with "2"]
- [find "C" in <name> and replace with "3"]
This set of three functions would change a file named "A ted's B file C" into "1 ted's 2 file 3".
Function Index
Click any function name to jump to its definition and examples.
[and (first expression), (second expression), …] | |
Definition | Returns true if all of the parameter expressions' lengths are greater than zero. This function is primarily designed to work with the [if] function. You will rarely (if ever) want to actually include the result in a new file name. |
Examples |
|
→ Function Index | |
[capitalize (expression)] | |
Definition | Returns a literal with the first character from each word in (expression) changed to its corresponding uppercase value, and all remaining characters set to their corresponding lowercase values. |
Examples |
|
→ Function Index | |
[concatenate (first expression), (second expression), …] | |
Definition | Returns a literal that contains all parameter expressions. |
Example |
|
→ Function Index | |
[created (strftime-style format)] | |
Definition | Returns the file's creation date, as formatted by (strftime-style format) |
Examples |
|
→ Function Index | |
[decrement (value to decrement), (value to decrement by)] | |
Definition | Decreases a value by one (default) or by value to decrement by, if defined. |
Examples |
|
→ Function Index | |
[equal (first expression), (second expression)] | |
Definition | Returns true if (first expression) and (second expression) are equal, otherwise returns an empty string constant. This function is primarily designed to work with the [if] function. You will rarely (if ever) want to actually include the result in a new file name. |
Examples |
|
→ Function Index | |
[find (query expression), (source expression), (replacement expression)] | |
Definition | Returns a constant with all occurrences of (query expression) in (source expression) replaced by (replacement expression). Put an (ignore case) statement somewhere near the end of the function to ignore the source expression's case. |
Examples |
|
→ Function Index | |
[findRegularExpression (query regular expression), (source expression), (replacement expression)] | |
Definition |
Returns a constant with all matches to (query regular expression) in (source expression) replaced by (replacement expression). Put an (ignore case) statement somewhere near the end of the function to ignore the source expression's case. Marked subexpressions can be interpolated into the replacement string using the syntax $n where n is the index of the subexpression, starting at 1. This replaces the \1, \2, etc. construct that you might know from other regular expression implementations. |
Example |
|
→ Function Index | |
[if (condition expression), (primary return expression), (secondary return expression)] | |
Definition | Returns (primary return expression) if (condition expression)'s length is greater than zero, otherwise returns (secondary return expression) |
Examples |
|
→ Function Index | |
[increment (value to increment), (value to increment by)] | |
Definition | Increases a value by one (default) or by value to increment by, if defined. |
Examples |
|
→ Function Index | |
[insert (insertion expression), (start index), (source expression)] | |
Definition |
Returns a constant where (insertion expression) has been inserted in (source expression) at (start index). Put a (backwards) statement somewhere near the end of the function to make (start index) count from (source expression)'s end instead of its beginning. Please note that although (start index) is numeric, all arguments must be surrounded by quotation marks. |
Examples |
|
→ Function Index | |
[letters from (starting value), (increment), (sleep value), (maximum index), (group identifier)] | |
Definition |
Returns a character constant that represents the original file's position in a sequence of items, as described by the sorting order of your file list. The group identifier, if included, will group the filenames in separate sequences for different evaluated identifier values, as opposed to simply incrementing the sequence number for all files. See the example in the Sequence section. You can omit parameters to have Name Mangler fill in default values. Please note that all arguments (including numeric ones) must be surrounded by quotation marks. The following examples assume that there is a total of 10 files in your file list. |
Examples |
(For examples demonstrating the effects of the other parameters, see [sequence].) |
→ Function Index | |
[lowercase (expression)] | |
Definition | Returns a constant with each character from (expression) changed to its corresponding lowercase value. |
Example |
|
→ Function Index | |
[modified (strftime-style format)] | |
Definition | Returns the file's modification date, as formatted by (strftime-style format). |
Examples |
|
→ Function Index | |
[not (expression)] | |
Definition | Returns true if (expression) is empty, otherwise returns an empty string constant. This function is primarily designed to work with the [if] function. You will rarely (if ever) want to actually include the result in a new file name. |
Examples |
|
→ Function Index | |
[now (strftime-style format)] | |
Definition | Returns the present date, as formatted by (strftime-style format). |
Examples |
|
→ Function Index | |
[or (first expression), (second expression), …] | |
Definition | Returns true if at least one of the parameter expressions' lengths is greater than zero. This function is primarily designed to work with the [if] function. You will rarely (if ever) want to actually include the result in a new file name.. |
Examples |
|
→ Function Index | |
pad (source expression), (length), (padding expression)] | |
Definition |
Returns a constant formed from (source expression) by either removing characters from the end, or by appending as many occurrences of (padding expression) as necessary. If you enter a negative value for (length), the padding will be added at the beginning of (source expression). Please note that although (length) is numeric, all arguments must be surrounded by quotation marks. |
Examples |
|
→ Function Index | |
[prompt …] | |
Definition | Returns a string that is entered by the user upon performing the renaming operation (this is most useful for Droplets). You can specify instructions for Name Mangler to show when prompting for input. Identical instructions will lead to the same string being used. |
Examples |
|
→ Function Index | |
[random (number of characters), (population of characters)] | |
Definition |
Returns a random string of length (number of characters) selected from (population of characters) Please note that all arguments (including numeric ones) must be surrounded by quotation marks. |
Examples |
|
→ Function Index | |
[remove (length), (start index), (source expression)] | |
Definition |
Returns a constant where a total of (length) characters at most have been removed from (source expression), starting at (start index). Put a (backwards) statement somewhere near the end of the function to make (start index) and (length) count from (source expression)'s end instead of its beginning. Please note that all arguments (including numeric ones) must be surrounded by quotation marks. |
Examples |
|
→ Function Index | |
[romanNumerals (start number), (increment), (sleep value), (maximum index), (group identifier)] | |
Definition |
Returns a roman numeral literal that represents the original file's position in a sequence of items, as described by the sorting order of your file list. The group identifier, if included, will group the filenames in separate sequences for different evaluated identifier values, as opposed to simply incrementing the sequence number for all files. See example in the Sequence section. You can omit parameters to have Name Mangler fill in default values. Please note that all arguments (including numeric ones) must be surrounded by quotation marks. The following examples assume that there is a total of 10 files in your file list. |
Examples |
(For examples demonstrating the effects of the other parameters, see [sequence].) |
→ Function Index | |
[sequence (starting index), (step value), (sleep value), (maximum index), (minimum number of digits), (group identifier)] | |
Definition |
Returns a literal that represents the original file's position in a sequence of items, as described by the sorting order of your file list. The group identifier, if included, will group the filenames in separate sequences for different evaluated identifier values, as opposed to simply incrementing the sequence number for all files. See example below. You can omit parameters to have Name Mangler fill in default values. Please note that all arguments (including numeric ones) must be surrounded by quotation marks. The following examples assume that there is a total of 10 files in your file list. |
Examples |
|
→ Function Index | |
[term (first expression), (second expression), …] | |
Definition |
Returns the parameter expression at the index that represents the original file's position in a sequence of items, as described by the sorting order of your file list. The following example assumes that there is a total of 10 files in your file list. |
Example |
|
→ Function Index | |
[titlecase (expression), (length cutoff)] | |
Definition |
Returns a literal with (expression) set to title case (first letter capitalized, plus the first letter of each word that's greater than (length cutoff) characters long. Words shorter than (length cutoff) will be converted to lowercase by default. To prevent this, add (lazy) after the cutoff value, as seen in the example below, and they will retain their original case. Please note that — although (length cutoff) is numeric — all arguments must be surrounded by quotation marks. |
Examples |
|
→ Function Index | |
[trim (expression)] | |
Definition | Returns a literal with leading and trailing spaces in (expression) removed. |
Example |
|
→ Function Index | |
[truncate (source expression), (maximum length)] | |
Definition |
Returns a literal with (source expression) truncated to (maximum length) characters if (source expression) is longer than (maximum length). Returns the original (source expression) otherwise. If a negative (maximum length) is used, truncation occurs from the start of (source expression). Please note that — although (maximum length) is numeric — all arguments must be surrounded by quotation marks. |
Examples |
|
→ Function Index | |
[uppercase (expression)] | |
Definition | Returns a literal with each character from (expression) changed to its corresponding uppercase value. |
Example |
|
→ Function Index |
So why is there a massively-large empty space here, with nothing else around it? Basically, it's some white space to help insure that the Quick Access entries at the top of the help window can properly highlight the entries at the bottom of the functions table. |